project
Code a Mars Rover Driving Game
Materials
1. Learn how rovers drive on Mars
Find out we safely navigate rovers around Mars from all the way back on Earth in this 60-second video. Credit: NASA/JPL-Caltech
Landing on the Red Planet is only the very first step in the life of a Mars rover. Once on the ground, the rover can begin its scientific journey as part of what we call surface operations.
This includes driving to points of interest, collecting images, using tools to make measurements and collect scientific data, as well as sending status reports back to Earth. However, the harsh terrain on Mars with hazards like boulders, sand, and craters means that moving rovers from one place to another is no simple task.
To get rovers from one place to another safely, engineers carefully plan out the routes they want a rover to take. Each day, they send code to the rover telling it where to go, how to get there, and which tasks to perform once it arrives.
In this activity you'll use code to design a game inspired by the way NASA rovers navigate on Mars.
2. Get familiar with pygame
- Begin by creating a backdrop space where your game will take place. Sample code is provided below.
- Running this code won’t do much but pop up a blank display screen. We’ve got some work to do before we have a working game!
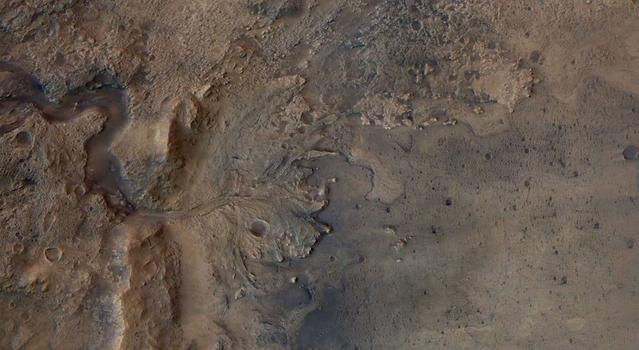
3. Set up the game environment
Before you start coding the game itself, you'll need to build the environment where the game will take place and begin to create rules for how that environment will operate.
Tasks:
- It may help to make a Game class that contains all your rules. Example code is provided below, but feel free to explore any code that feels most comfortable.
- Within the same __init__ in the Game class, we also want to start decorating our game. Include a background image, such as one of those provided in the materials list, to replace the plain white background.
- Test your code to this point to confirm you aren’t getting any errors, although we’re still not generating much yet.
- Introduce your background and the commands to quit or close the game. Test your code to confirm you can generate a game window with a Martian landscape of your choosing.
About the image: This image of Jezero Crater on Mars was taken by the European Space Agency's Mars Express Orbiter and shows the remains of an ancient delta. NASA's Perseverance Mars rover landed in Jezero Crater in February 2021 and is now exploring the area with a suite of advanced science instruments. › Full image and caption
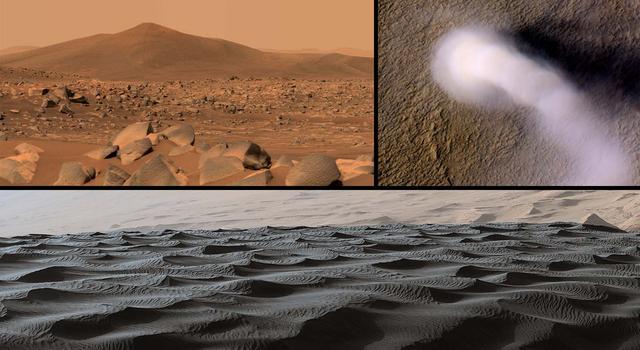
4. Introduce the game objects
On Mars, rovers have to navigate hazards such as rocky or sandy terrain, dust storms, and other inclement weather.
Now that we have set up our game environment, we’re ready to add our rover and the hazards players will need to avoid.
Tasks:
- Download the Mars rover sprite from the materials list to use as your rover – or use your own image or graphic.
- Now, create a destination that the rover will need to reach to succeed in its mission.
- Create and place as many hazards as you’d like within your game using images and graphics of your choice.
- Remember to give your code a test at each step!
About the images: Hazards are everywhere on Mars and can include rocky terrain, dust devils, and rippled sand dunes, as shown in these images (clockwise from left) taken on and at Mars by the Perseverance rover, Mars Reconnaissance Orbiter, and Curiosity rover, respectively. | + Expand image
5. Get your game objects moving
At this point, we have placed our rover on the Martian terrain and created the hazards it must avoid, but at this point, they are all stationary. We need to write rules that move our objects around the game environment. This is where pygame starts to come in handy.
Tasks:
- Build a game loop that will determine the rules for when the game is active and how your rover will move. Explore how pressing or releasing keys can be used to drive your rover.
- It may help to put the rover and the hazards, along with their rules for moving, in one loop, but this can be done across multiple loops or even across multiple libraries. For simplicity, an example of putting them in one place is provided below, but note that this is not the most efficient approach.
- After each movement, we need to be sure the screen refreshes. (Although we don’t see it, the screen is refreshing constantly.) That is to say, we want the game loop to run after every single movement, not just move once and hang.
- Include movement commands for your hazards as well. Be sure that the hazards don’t move beyond the game environment!
- Be creative to make the game your own. For example, you can add additional objects or hazards to make your game as hard as you’d like. You can also introduce levels into your game, adding hazards that are more challenging to overcome as the levels progress.
- Determine how the mission will be deemed a success (the rover reaches the target) or a failure (the rover collides with a hazard).
- Close your For loop with a graphics update, otherwise the game will hang upon completing each of the steps above. This is also a good time to include level-ups in your While loop.
About the image: This interactive map shows the landing site and movements of NASA's Perseverance rover within Jezero Crater. Perseverance landed on Feb. 18, 2021. The map also shows the location of the Mars Helicopter. | › Learn more on the NASA Mars Exploration website
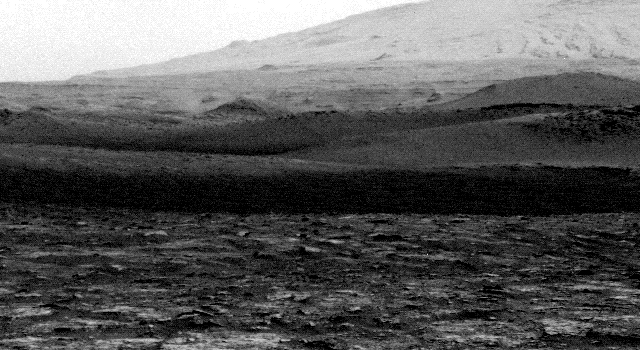
6. Define the player and the hazard
When driving Mars rovers, we have to be very intentional about how we move a rover across the harsh landscape to reach its destination. This means that the best path is not always the shortest path as we may need to evade treacherous areas or conditions. While the rover is meticulously monitored and controlled, the dangerous conditions on Mars can’t always be avoided.
In the previous step, we set our game objects in motion, but our rover and the hazards should be moving differently.
Tasks:
- Next, you will need to define the rules for a GameObject (such as the rover and hazards) using .self and parameters of your choosing (image_path, height, width, speed, etc.). Tinker with these rules as you see fit to make the game more interesting for you!
- First, we will define the properties specific to our rover, including how it moves and what happens if it collides with a hazard. Again, this can be imported from a separate file for cleanliness if preferred.
- Observe how in this example, height is used to be sure the rover never drives off the game window.
- Consider the kinds of hazards we might encounter on Mars, how they would behave, and how we might be alerted to their presence as you complete this next step and further define the behavior of hazards in your game. In our first pass, hazards had a fairly simple set of parameters. Feel free to add more complexities to them as you see fit.
About the image: This gif of a dust devil on Mars was created from images captured by NASA's Curiosity rover in August 2020. The dust plume disappears past the top of the frame, so an exact height can't be known, but it's estimated to be at least 164 feet (50 meters) tall. | › Full image and caption
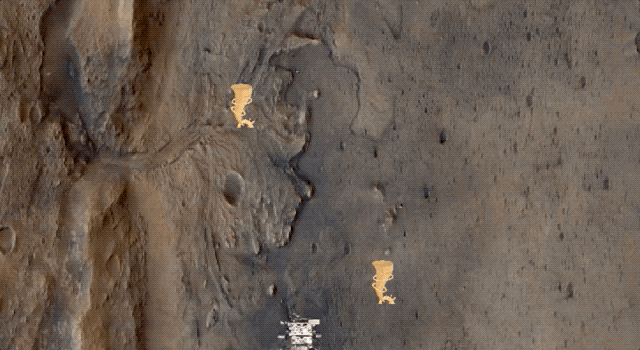
7. Put it all together!
Hopefully you have been testing your code at each step to identify any typos and issues around indentation. It can be tough to keep track of how you want your loops to interact. Coding in Python is all about troubleshooting and learning from your mistakes, so use any traceback errors as hints to guide you as you track down your bugs. In the end, you’ll have created a game inspired by NASA Mars exploration!
+ Expand image
8. Take it farther
When playing the game, think about how you do as you progress through the levels. Is it too hard or too easy? Think about ways to refine your game not just in terms of difficulty, but also complexity. For example, did you increase the number of hazards as the game levels progress? What about introducing movement in more directions for both you and your rover?
Also, check out this Mars rover game from NASA for more inspiration.